User case: In some situations, user may want to see only few fields in his output of the ALV or he may want to see different version of layout from the one being displayed. This blog post explains the approach to achieve the requirement using Factory Method.
Let us assume the following query where Material details from tables MARA (Material Master), MAKT (Material Description) and MARC( Plant Data for Material ) as shown below.
DATA:lr_alv TYPE REF TO cl_salv_table.
SELECT a~matnr, a~mtart, a~matkl, a~meins,
b~werks,
b~ekgrp,
c~maktx,
c~spras
FROM mara AS a INNER JOIN marc AS b
ON a~matnr = b~matnr
INNER JOIN makt AS c
ON a~matnr = c~matnr
INTO TABLE @DATA(lt_output)
UP TO 100 ROWS.
DELETE ADJACENT DUPLICATES FROM lt_output COMPARING ALL FIELDS.
CALL METHOD cl_salv_table=>factory
IMPORTING
r_salv_table = lr_alv " Basis Class Simple ALV Tables
CHANGING
t_table = lt_output.
The output is as shown:
The ALV output does not have any of the sort, filter or other such options when called using factory method. To get them, add the following code before calling the display method.
DATA: gr_functions TYPE REF TO cl_salv_functions.
DATA: gr_display TYPE REF TO cl_salv_display_settings.
** To Display the Sort, Filter Export, Etc,. options
gr_functions = lr_alv->get_functions( ).
gr_functions->set_all( abap_true ).
gr_display = lr_alv->get_display_settings( ). "Display Settings
* Header
gr_display->set_list_header( 'Material Details ' ). " Heading for the ALV
* Zebra pattern
gr_display->set_striped_pattern( abap_true ). " To set the striped Pattern
* Output
lr_alv->display( ).
Now the output is shown with Sort, Filter, Export, etc.,
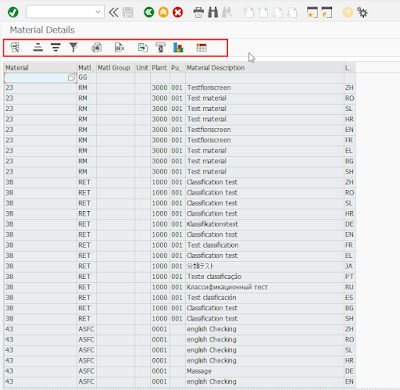
Observe that the option to save the layout is still not available. Calling the following methods before calling display would do that.
DATA: lv_key TYPE salv_s_layout_key.
* Saving Layouts.
lv_key-report = sy-repid.
gr_layout->set_key( lv_key ).
gr_layout->set_save_restriction( cl_salv_layout=>restrict_none ).
Calling the above methods allows user to Save the Layouts and select the saved layouts when needed. The Icons will be as shown below.
Using them, We can alter the ALV Layout and Save that layout by giving some name to it.
I have applied a sort and a filter in the below screenshot.
Click on the Save layout Icon. Provide the values to the Layout and Name and save it.
Hence, the different versions of layouts can be obtained in a go without altering it every time. The saved layouts are displayed as shown.
The saved layouts can be added to the selection screen as F4 Help and a specific layout can be set as default. Add the following code for that. It provides the values of Saved layout in the F4 Help.
DATA:set_lay TYPE slis_vari,
g_exit TYPE c,
g_variant TYPE disvariant.
g_variant-report = sy-repid.
CALL FUNCTION 'REUSE_ALV_VARIANT_F4'
EXPORTING
is_variant = g_variant
i_save = 'A'
IMPORTING
e_exit = g_exit
es_variant = g_variant
EXCEPTIONS
not_found = 1
program_error = 2
OTHERS = 3.
.
IF sy-subrc = 0.
p_var = g_variant-variant.
set_lay = g_variant-variant.
ENDIF.
gr_layout->set_initial_layout( value = set_lay ).
lr_alv->display( )..
Selection screen: I have created ALV layouts from the output screen. They are shown in the F4 Help now. Selecting a layout would display its output with that layout.
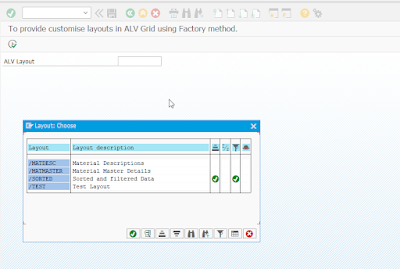
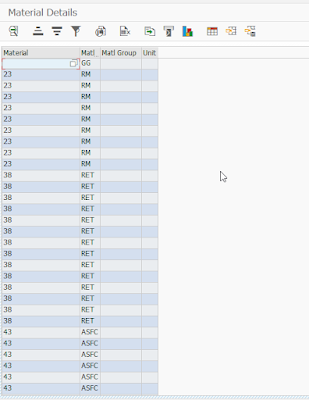
User can also switch between layouts from output screen itself using ” Select layout icon.
The Report code is below.
REPORT zmt_customlayout_factory.
PARAMETERS: p_var TYPE disvariant-variant.
DATA:lr_alv TYPE REF TO cl_salv_table.
DATA: gr_layout TYPE REF TO cl_salv_layout, "cl_salv_table,
lv_key TYPE salv_s_layout_key,
gr_table TYPE REF TO cl_salv_table.
DATA: gr_functions TYPE REF TO cl_salv_functions.
DATA: gr_display TYPE REF TO cl_salv_display_settings.
DATA: gr_columns TYPE REF TO cl_salv_columns_table.
DATA: gr_column TYPE REF TO cl_salv_column_table.
DATA: gr_sorts TYPE REF TO cl_salv_sorts.
DATA: gr_agg TYPE REF TO cl_salv_aggregations.
DATA:set_lay TYPE slis_vari,
g_exit TYPE c,
g_variant TYPE disvariant.
AT SELECTION-SCREEN ON VALUE-REQUEST FOR p_var.
g_variant-report = sy-repid.
CALL FUNCTION 'REUSE_ALV_VARIANT_F4'
EXPORTING
is_variant = g_variant
i_save = 'A'
IMPORTING
e_exit = g_exit
es_variant = g_variant
EXCEPTIONS
not_found = 1
program_error = 2
OTHERS = 3.
.
IF sy-subrc = 0.
p_var = g_variant-variant.
set_lay = g_variant-variant.
ENDIF.
START-OF-SELECTION.
SELECT a~matnr, a~mtart, a~matkl, a~meins,
b~werks,
b~ekgrp,
c~maktx,
c~spras
FROM mara AS a INNER JOIN marc AS b
ON a~matnr = b~matnr
INNER JOIN makt AS c
ON a~matnr = c~matnr
INTO TABLE @DATA(lt_output)
UP TO 100 ROWS.
DELETE ADJACENT DUPLICATES FROM lt_output COMPARING ALL FIELDS.
END-OF-SELECTION.
CALL METHOD cl_salv_table=>factory
IMPORTING
r_salv_table = lr_alv " Basis Class Simple ALV Tables
CHANGING
t_table = lt_output.
** To Display the Sort, Filter Export, Etc,. options
gr_functions = lr_alv->get_functions( ).
gr_functions->set_all( abap_true ).
gr_display = lr_alv->get_display_settings( ). "Display Settings
* Header
gr_display->set_list_header( 'Material Details ' ). " Heading for the ALV
* Zebra pattern
gr_display->set_striped_pattern( abap_true ). " To set the striped Pattern
* Layout changes
gr_columns = lr_alv->get_columns( ).
gr_sorts = lr_alv->get_sorts( ).
gr_agg = lr_alv->get_aggregations( ).
gr_layout = lr_alv->get_layout( ).
* Saving Layouts.
lv_key-report = sy-repid.
gr_layout->set_key( lv_key ).
gr_layout->set_save_restriction( cl_salv_layout=>restrict_none ).
* Setting layout screen from Selection screen.
gr_layout->set_initial_layout( value = set_lay ).
lr_alv->display( )..
Source: sap.com
No comments:
Post a Comment